Computer graphics and 3D rendering have become essential aspects of various fields, ranging from video games and animation to architecture and industrial design. As a student seeking help with image processing assignment using python in computer science, computer graphics, or related fields, you may often encounter assignments that require you to implement 3D rendering techniques. One of these techniques is the Scanline Algorithm, which is a fundamental method for rendering 3D images. In this comprehensive guide, we will explore the Scanline Algorithm and demonstrate how to implement it using Python. By the end of this tutorial, you will have a clear understanding of the algorithm, its application, and the necessary skills to tackle university assignments involving 3D image rendering. Setting up your Python environment is the first step in this exciting journey. You'll need a development environment with the necessary libraries and tools. This involves installing Python, along with libraries like NumPy and Matplotlib for handling numerical operations and visualizing results. With your environment set up, you can proceed to build a simple 3D scene. This involves defining the vertices of a cube and specifying its faces by their vertex indices. You'll also create a 2D canvas using NumPy, which will serve as the rendering surface for your 3D scene. Once your scene is set, it's time to dive into implementing the Scanline Algorithm, a crucial part of this guide.
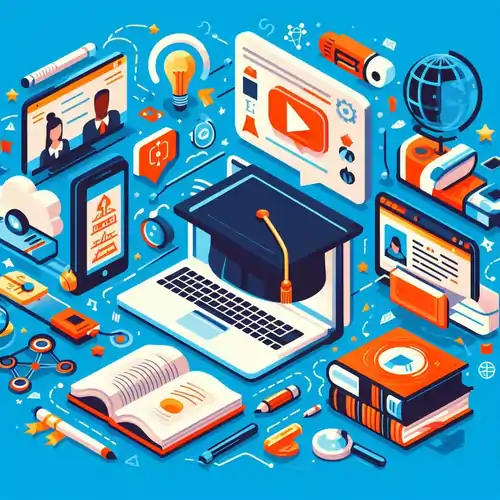
The algorithm works by scanning each horizontal line or row of pixels in the 2D rendering surface, identifying intersections with polygon edges, and determining pixel colors while considering factors like shading and texturing. We'll provide you with code snippets to help you understand the implementation better. Additionally, we'll discuss the importance of shading and texturing to enhance the realism of your 3D renders, introducing you to advanced concepts like Phong and Gouraud shading and texture mapping. We'll also touch upon the complexities of handling more intricate 3D scenes with multiple objects, lighting effects, and occlusion. While this guide provides a strong foundation for tackling 3D rendering assignments, the world of computer graphics is vast and continually evolving. Therefore, we'll conclude by suggesting avenues for further learning, emphasizing the need to stay organized, practice debugging, seek help and collaborate, explore 3D libraries, work on real projects, and stay informed about the latest trends in the field. By following this comprehensive guide and embracing these recommendations, you'll be well-prepared to excel in your university assignments and embark on an exciting journey in the world of 3D rendering and computer graphics.
Understanding 3D Rendering
Understanding 3D rendering is fundamental in the modern digital landscape. It serves as the bridge between the virtual and real worlds, encompassing a range of technologies that create lifelike, immersive experiences across various fields. At its core, 3D rendering is the process of transforming three-dimensional data into two-dimensional images, enabling us to visualize complex objects, scenes, and animations. In the context of computer graphics, this transformation involves simulating how light interacts with objects in a 3D space and projecting the resulting image onto a 2D surface, typically a computer screen. Whether you're a student embarking on a computer science or design-related program or a professional in fields like video game development, architecture, or film production, understanding 3D rendering is crucial. It empowers you to harness the power of visualization and storytelling, offering the ability to create virtual worlds, architectural designs, special effects, and interactive simulations. The process involves various techniques and algorithms, each tailored to specific needs, from real-time rendering for gaming to photorealistic rendering for movies. In the journey to comprehend 3D rendering, one encounters essential concepts like modeling, shading, texturing, and illumination, which collectively breathe life into 3D scenes. As you delve deeper into this subject, you'll explore topics like ray tracing, which simulates the behavior of light rays to achieve stunning visual realism, and rasterization, a technique used for rapid, real-time rendering in video games. Additionally, understanding the hardware and software tools that facilitate 3D rendering, such as graphics processing units (GPUs) and rendering engines, becomes essential. Whether you aim to master the intricacies of shader programming, discover the secrets of global illumination, or simply want to appreciate the magic behind your favorite animated film or video game, a solid grasp of 3D rendering is the gateway to unlocking a world of creativity and innovation. It's the art and science of making pixels come alive, enabling you to bring your wildest digital dreams to life.
Implementation of Scanline Algorithm
- Introduction to the Scanline Algorithm: The Scanline Algorithm is a pivotal method in the field of computer graphics, specifically designed for rendering 3D images. It operates by systematically scanning each horizontal line or row of pixels in a 2D rendering surface, such as a computer screen, and determining the intersections with the edges of polygons within the 3D scene.
- Sorting Edges by Y-Coordinate: A crucial initial step in the Scanline Algorithm involves sorting the edges of the polygons by their y-coordinates. This sorting enables the algorithm to identify the starting and ending points of each scanline, ensuring an organized approach to rendering.
- Creating a List of Active Edges: For every scanline, the algorithm assembles a list of active edges. These active edges are those that intersect with the scanline at that specific y-coordinate, and they are critical in determining which parts of the polygon are visible on the screen.
- Traversal of Active Edges: The algorithm traverses the list of active edges for the current scanline, carefully filling in the pixels between these edges. During this process, it factors in shading and texturing, which contribute to the visual realism of the 3D scene.
- Iterative Process: The scanline-by-scanline approach continues until the entire image is rendered. Each iteration, which corresponds to a horizontal row of pixels, contributes to the final rendering of the 3D scene. This iterative nature ensures that the entire scene is accurately portrayed on the 2D rendering surface.
- Handling Complex Scenes: The Scanline Algorithm is versatile and can handle complex scenes with multiple polygons, intricate shapes, and varying shading. It is particularly adept at rendering surfaces with varying levels of detail, making it a valuable tool for computer graphics professionals working on 3D games, animations, architectural visualization, and more.
- Enhancements and Advanced Techniques: While the basic Scanline Algorithm provides a strong foundation for rendering 3D scenes, professionals often incorporate enhancements and advanced techniques to achieve superior results. These may include more sophisticated shading models, texture mapping, and optimizations for real-time rendering.
- Role in Real-Time Graphics: In addition to its role in offline rendering, the Scanline Algorithm is essential in real-time graphics, such as video games. Its efficiency in rendering complex scenes in real-time environments makes it a key component of modern gaming engines.
- Continual Evolution: The Scanline Algorithm is part of the rich history of computer graphics, and its concepts continue to evolve as technology advances. New rendering techniques, hardware acceleration, and software improvements keep the field dynamic and ever-changing.
Setting Up Your Python Environment
To begin implementing the Scanline Algorithm in Python, you'll need a development environment with the necessary libraries and tools. Below are the steps to set up your environment:
Python Installation: Ensure you have Python installed on your computer. You can download the latest version from the official Python website (https://www.python.org/downloads/).
Required Libraries: You'll need a few libraries to assist with graphics and visualization. The most important ones are NumPy for numerical operations and Matplotlib for visualizing the results. You can install these using pip:
pip install numpy matplotlib
Integrated Development Environment (IDE): Choose a Python IDE or code editor that you are comfortable with. Some popular options include PyCharm, Visual Studio Code, and Jupyter Notebook.
With your environment set up, you're ready to start implementing the Scanline Algorithm.
Building a Simple 3D Scene
Before we dive into the algorithm itself, let's create a basic 3D scene that we can use as the canvas for rendering. We'll use a simple list of vertices and polygons to represent the scene. For this example, we'll render a cube. Here's a basic Python script to set up the scene:
import numpy as np
import matplotlib.pyplot as plt
# Define vertices of the cube
vertices = np.array([
[0, 0, 0],
[1, 0, 0],
[1, 1, 0],
[0, 1, 0],
[0, 0, 1],
[1, 0, 1],
[1, 1, 1],
[0, 1, 1]
])
# Define faces (polygons) of the cube by specifying vertex indices
faces = [
[0, 1, 2, 3], # Bottom face
[4, 5, 6, 7], # Top face
[0, 1, 5, 4], # Front face
[2, 3, 7, 6], # Back face
[0, 3, 7, 4], # Left face
[1, 2, 6, 5] # Right face
]
# Create a 2D canvas for rendering
canvas = np.zeros((400, 400, 3), dtype=np.uint8)
# Visualize the cube (for testing)
plt.imshow(canvas)
plt.show()
Implementing the Scanline Algorithm
Now that we have our 3D scene set up, we can move on to implementing the Scanline Algorithm to render the cube. The code for implementing the algorithm is as follows:
def draw_scanline(canvas, y, x1, x2, z1, z2, color):
# Check for out-of-bounds coordinates
if y < 0 or y >= canvas.shape[0]:
return
# Ensure x1 is to the left of x2
if x1 > x2:
x1, x2 = x2, x1
z1, z2 = z2, z1
# Clip to the canvas boundaries
x1 = max(0, min(x1, canvas.shape[1] - 1))
x2 = max(0, min(x2, canvas.shape[1] - 1))
# Calculate the delta values for z and color components
delta_z = (z2 - z1) / (x2 - x1)
delta_color = [(color[1] - color[0]) / (x2 - x1),
(color[2] - color[1]) / (x2 - x1),
(color[3] - color[2]) / (x2 - x1)]
# Initialize z and color
z = z1
color_val = color[0]
# Iterate over pixels in the scanline
for x in range(int(x1), int(x2) + 1):
# Check for z-buffering (only draw if z is closer)
if z < canvas[y, x, 0]:
# Update the z-buffer
canvas[y, x, 0] = z
# Update the pixel color
canvas[y, x, 1:] = color_val
# Update z and color for the next pixel
z += delta_z
color_val += delta_color
def render_scanline(canvas, vertices, faces):
# Initialize the z-buffer with positive infinity
canvas[:, :, 0] = np.inf
# Iterate over each face (polygon)
for face in faces:
# Extract vertex positions and colors
vertices_face = vertices[face]
colors = np.random.rand(4) # Random color for testing
# Loop through the vertices to render the edges
for i in range(4):
x1, y1, z1 = vertices_face[i]
x2, y2, z2 = vertices_face[(i + 1) % 4]
draw_scanline(canvas, int(y1), x1, x2, z1, z2, colors)
# Call the rendering function
render_scanline(canvas, vertices, faces)
# Visualize the rendered cube
plt.imshow(canvas[:, :, 1:])
plt.show()
In the code above, we've implemented the draw_scanline function, which is responsible for rendering a horizontal scanline by interpolating color values and performing z-buffering. The render_scanline function iterates over the faces of the cube, extracts the vertices and random colors for testing, and then calls draw_scanline for each edge of the face.
Shading and Texturing
To enhance the realism of your rendered 3D objects, you can implement shading and texturing techniques. Shading techniques like Phong shading or Gouraud shading can be applied to achieve smooth lighting effects, and texture mapping can be used to map images onto the surfaces of 3D objects. These techniques can be complex and involve more in-depth knowledge of computer graphics. However, they can significantly improve the quality of your 3D renders.
Handling Complex Scenes
While the code presented here is a simplified example, the Scanline Algorithm can be applied to more complex 3D scenes with multiple objects, textures, and lighting effects. Handling complex scenes often requires data structures for efficient organization and algorithms for handling occlusion, transparency, and other factors. To tackle more advanced projects and assignments, it's important to explore additional topics in computer graphics.
Conclusion and Further Learning
In this comprehensive guide, we've introduced you to the Scanline Algorithm, a fundamental technique for rendering 3D images, and demonstrated how to implement it using Python. By following the steps and examples provided, you should now have a solid foundation for working on university assignments related to 3D rendering.
To deepen your understanding and explore more advanced topics in computer graphics, consider the following avenues for further learning:
- Study advanced shading techniques like Phong and Gouraud shading.
- Explore texture mapping and UV mapping for realistic texturing.
- Learn about ray tracing, a popular rendering technique for photorealistic images.
- Experiment with 3D modeling software and file formats like OBJ and STL.
- Consider taking a course or reading textbooks on computer graphics and rendering.
As you progress in your studies and gain experience, you'll be better equipped to handle more complex 3D rendering assignments and projects. The world of computer graphics is vast and continually evolving, offering exciting opportunities for creative and technical exploration.
Conclusion
In this comprehensive guide, we've explored the scanline algorithm for 3D rendering in Python, providing a solid foundation for students and enthusiasts looking to delve into the world of computer graphics. We started by creating a 3D scene, implemented perspective projection, and then used the scanline algorithm to render the scene while applying flat shading. To continue your journey in 3D rendering and computer graphics, consider exploring advanced rendering techniques, real-time rendering, and GPU programming. Additionally, you can study industry-standard libraries and frameworks, such as OpenGL, DirectX, and WebGL, to gain a deeper understanding of how 3D rendering is applied in practice. As you work on assignments and personal projects, you'll develop valuable skills that can open up exciting opportunities in the ever-evolving field of computer graphics. Happy rendering!