In the dynamic landscape of computer science and programming, Python emerges as an exceptionally versatile language, proficient in tackling a myriad of domains. Particularly for students engaged in university assignments that bridge the realms of technology and art, undertaking a project involving the creation of a program to display art titles based on artist names presents a compelling venture. The significance of such a project lies not only in its alignment with the academic curriculum but also in its potential to fortify and elevate students' programming acumen. This blog aspires to serve as a comprehensive guide, ushering students through the intricate process of developing such a program in Python. Delving into the intricacies of the task at hand, this guide encompasses elucidative step-by-step explanations, snippets of code that exemplify best practices, and pragmatic tips garnered from real-world applications. The intention is not merely to facilitate the accomplishment of assignment requirements but to instill a deeper understanding of Python's capabilities and applications, thereby nurturing a more profound mastery of programming skills. As students navigate through the various stages of this programming endeavor, from conceptualization to execution, they are encouraged to grasp the underlying principles and methodologies. The chosen dataset structure, in this instance a dictionary mapping artist names to lists of associated art titles, serves as a pedagogical instrument, illustrating practical data handling. Beyond the rudimentary aspects of the code, the blog advocates for a nuanced approach to problem-solving by introducing additional features to the program. Suggestions such as case-insensitive search capabilities and partial name matching not only enhance the program's robustness but also illuminate the iterative and adaptive nature of programming practices. Importantly, the program is positioned as a foundation upon which students can build, encouraging exploration into more advanced topics like interfacing with external data sources or implementing graphical user interfaces. Through the lens of this Python-based art title display program, students are not only equipped with a functional tool for their immediate assignment needs but are also empowered to extrapolate and apply their newfound skills in diverse programming contexts. Thus, the blog endeavors to help with your Python assignment and demystify the complexities of this particular programming task, fostering a holistic and experiential learning journey for students grappling with the intersection of technology and art in the academic arena.
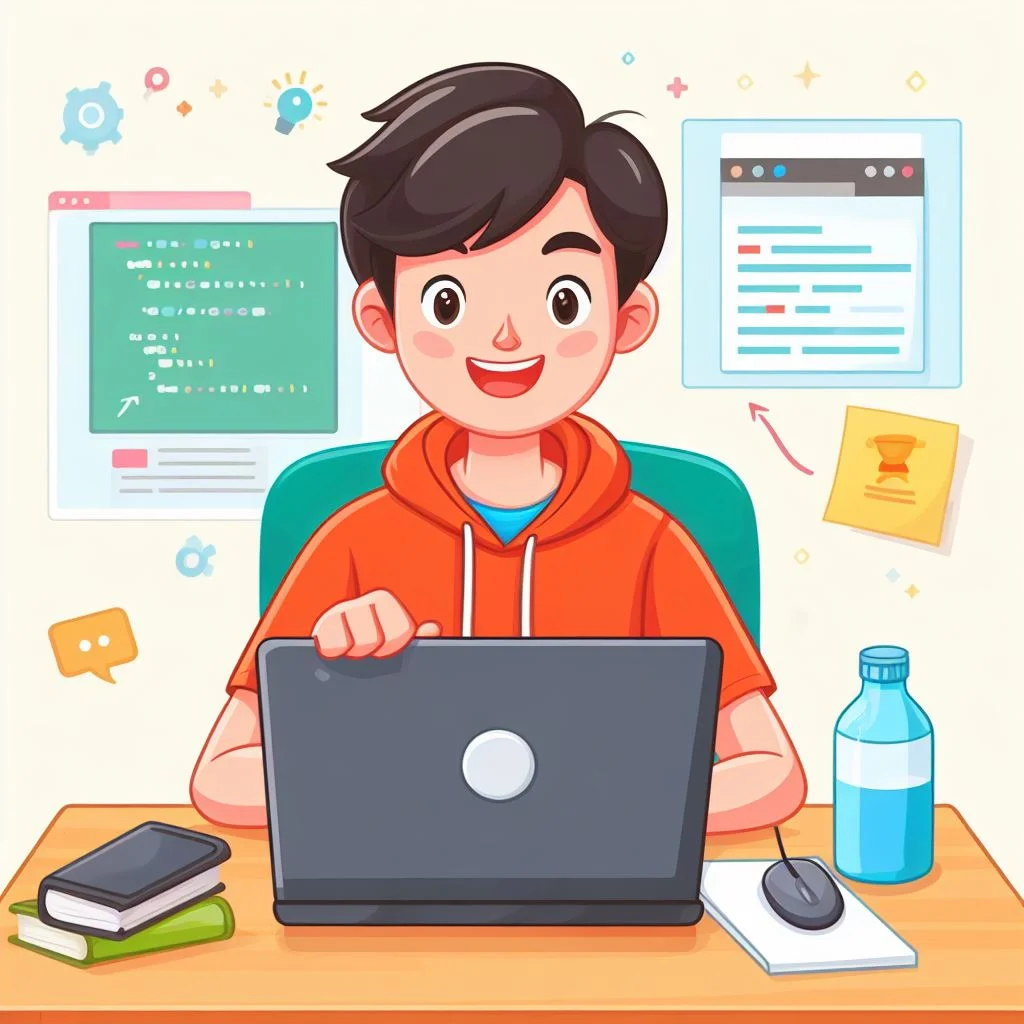
Understanding the Requirements
In the initial stages of crafting a solution, a foundational step entails a comprehensive grasp of the assignment's prerequisites. Prior to immersing oneself in the intricacies of code, it is imperative to discern the specific demands intrinsic to the given task. In the context delineated, the primary objective is evident: the development of a program capable of soliciting an artist's name as input and subsequently presenting a comprehensive catalog of art titles associated with that particular artist. The nuances concerning the data structure employed for storing this information, along with the origin of the data itself, introduce variables that may exhibit variability. To streamline the development process and facilitate clarity in explanations, a deliberate simplification is introduced—adopting a dictionary as the chosen data structure. In this construct, artist names are designated as keys, each corresponding to a list encapsulating their respective art titles. This deliberate abstraction not only expedites the comprehension of programming concepts but also serves as a foundational scaffold, allowing students to progressively advance towards more intricate data structures or diverse data sources as they refine their programming skills. Hence, a clear understanding of the assignment's requirements emerges as the linchpin, guiding the subsequent coding endeavors and ensuring a focused and effective response to the specific challenges posed by the task at hand. This approach underscores the strategic aspect of software development, encouraging students to not only generate code that meets immediate needs but also fostering a profound understanding of problem-solving methodologies applicable to diverse programming scenarios.
Components Required
To undertake the task of displaying art titles based on artist names in Python, you will need several essential components. First and foremost, Python should be installed on your system, serving as the foundational programming language for this project. Python's versatility and user-friendliness make it an ideal choice. Next, the BeautifulSoup library, a powerful web scraping tool, is necessary to parse and navigate HTML documents with ease. This enables precise extraction of artist names and artwork titles from web pages. The requests library is equally vital for making HTTP requests, streamlining web data retrieval. Moreover, you'll require access to a structured artist name and artwork database, sourced from websites, CSV files, or other structured data sources. This database forms the bedrock of your Python program, allowing you to associate artists with their respective titles. Meticulous curation of this database ensures accuracy and relevance throughout the project.
- Python: Before you embark on your journey to display art titles based on artist names, it's crucial to ensure you have Python installed on your system. If you haven't already, download and install Python from the official website (https://www.python.org/). Python is the backbone of our programming endeavor, providing a versatile and user-friendly platform for data manipulation and web interaction.
- Web Scraping Library: To effectively extract information from websites, we'll rely on Python's BeautifulSoup library. This powerful library allows us to parse and navigate HTML documents effortlessly. With BeautifulSoup, we can target specific elements on web pages, making the extraction of artist names and artwork titles a straightforward process.
- HTTP Requests Library: In the world of web scraping, the ability to retrieve web pages is paramount. We'll employ the requests library, an essential tool for making HTTP requests. This library streamlines the process of fetching data from the web, ensuring that we have access to the artist information we need.
- Artist Name and Artwork Database: A crucial component of this project is access to a reliable database containing artist names and their associated artworks. This database can be sourced from various places, such as a website with structured artist profiles and their works, a CSV file that you've prepared, or any other structured data source. A well-organized database forms the foundation of our Python program, enabling us to associate artists with their respective titles. Be sure to curate your database meticulously to ensure accuracy and relevancy.
Setting Up the Data
Initiating the data preparation phase involves the establishment of a sample dataset, commencing with the definition of data structure through a dictionary. This foundational step sets the stage for subsequent programming endeavors, offering a practical representation of how information will be organized and accessed. By employing a dictionary, keys correspond to artist names, while associated values form lists containing art titles. This simplified dataset serves as a fundamental illustration, providing clarity and a starting point for students to understand the relationship between data structures and programming logic in the context of their assignment.
artworks = {
'Leonardo da Vinci': ['Mona Lisa', 'The Last Supper', 'Vitruvian Man'],
'Vincent van Gogh': ['Starry Night', 'Sunflowers', 'The Bedroom'],
'Pablo Picasso': ['Guernica', 'Les Demoiselles d\'Avignon', 'The Weeping Woman']
# Add more artists and artworks as needed
}
This dictionary serves as a simplified representation of the data. In a practical scenario, the data might be sourced from external files, databases, or APIs, reflecting the diverse ways in which Python can interact with real-world data. This initial step is crucial, setting the stage for subsequent programming tasks where we'll manipulate and display this data based on user input.
Creating the Python Program
Embarking on the creation of the Python program, the next phase unfolds in the development process. The focal point is crafting a program designed to receive an artist's name as input and subsequently showcase the array of art titles linked to that specific artist. This step involves translating the conceptual framework into tangible code. By leveraging Python's syntax and functionality, the program brings the abstract idea to life, creating an interactive interface for users. The program's core functionality lies in its ability to access the dataset, locate the artist, and present the associated art titles, forming a crucial bridge between the conceptualization of the task and its practical implementation.
def display_art_titles(artist_name):
if artist_name in artworks:
titles = artworks[artist_name]
print(f"Art titles by {artist_name}:")
for title in titles:
print(f"- {title}")
else:
print(f"No information found for {artist_name}")
# Example usage:
user_input = input("Enter the artist's name: ")
display_art_titles(user_input)
This program follows a logical structure. It begins by checking if the entered artist's name exists in the artwork dictionary. If it does, it retrieves the associated art titles and prints them, providing the user with a visually appealing list. On the other hand, if the artist's name is not found in the dictionary, a message is displayed, informing the user that no information was found. The use of functions enhances code readability and reusability, contributing to good programming practices. As students delve into this code, they gain valuable insights into basic control flow structures and the importance of user input in designing interactive programs.
Improving the Code
Elevating the program's capabilities involves a strategic pursuit of enhancements to fortify its robustness and user-friendliness. Several key features can be implemented to augment the program's functionality. Firstly, introducing case-insensitive search enables users to input artist names without constraints, as the program uniformly handles varying cases. Secondly, incorporating partial name matching allows for flexibility, offering suggestions when users input incomplete artist names. These enhancements not only refine the user experience but also showcase the program's adaptability. By seamlessly integrating these features, the program transcends basic functionality, evolving into a sophisticated tool that caters to diverse user inputs and demonstrates a nuanced understanding of user interaction dynamics.
- Error Handling: Add error handling to deal with cases where the artist's name is not found in the dataset or when there are issues with the dataset file.
- Data Cleaning: Perform data cleaning and preprocessing to handle variations in artist names (e.g., uppercase/lowercase, misspellings) more effectively.
- Data Visualization: Create visualizations, such as bar charts or word clouds, to represent the art titles by each artist for a more engaging presentation in your assignments.
- Interactive User Interface: Develop a graphical user interface (GUI) for the application to make it user-friendly and visually appealing.
- Search and Filter: Add functionality to search for artists and filter artworks based on various criteria, such as time period or genre.
- Data Enrichment: Consider enriching your dataset with additional information, such as descriptions, genres, or historical context, to make your art analysis more comprehensive.
- Compatibility with Web Data: Extend the application to retrieve art data from online sources, such as art museums' websites, using web scraping techniques.
- Machine Learning: Explore machine learning techniques to predict artist names or art titles based on image analysis, if you have access to image data.
Case-insensitive Search
Implementing a case-insensitive search feature is a pivotal enhancement, fostering user flexibility and ease of interaction. By accommodating artist names entered in any case, the program ensures inclusivity and user-friendly functionality. This is achieved through a systematic approach of converting both the user input and the dictionary keys to lowercase, eradicating case discrepancies. Consequently, users can input artist names without concern for letter case, streamlining the search process and enriching the overall user experience. This enhancement reflects a keen awareness of user preferences, contributing to the program's adaptability and reinforcing its effectiveness in diverse usage scenarios.
def display_art_titles(artist_name):
artist_name = artist_name.lower()
if artist_name in artworks:
titles = artworks[artist_name]
print(f"Art titles by {artist_name.capitalize()}:")
for title in titles:
print(f"- {title}")
else:
print(f"No information
By converting both the input and dictionary keys to lowercase, the program ensures a case-insensitive search. This enhancement caters to user convenience, making the program more user-friendly and accommodating of different input styles.
Partial Name Matching
Enabling partial name matching stands as a pivotal augmentation, enhancing user interaction and expanding the program's versatility. This feature empowers users to input partial artist names, opening avenues for more flexible and intuitive searches. The program responds by providing suggestions based on the partial input, offering a responsive and user-centric experience. The implementation involves a systematic evaluation of input against available artist names, presenting potential matches. This not only caters to instances of uncertain or incomplete user inputs but also showcases the program's adaptability to varied search patterns. The incorporation of partial name matching elevates the program beyond basic functionality, embodying a nuanced responsiveness to user needs.
def display_art_titles(artist_name):
artist_name = artist_name.lower()
matches = [artist for artist in artworks.keys() if artist_name in artist.lower()]
if not matches:
print(f"No matches found for {artist_name.capitalize()}")
elif len(matches) == 1:
matched_artist = matches[0]
titles = artworks[matched_artist]
print(f"Art titles by {matched_artist.capitalize()}:")
for title in titles:
print(f"- {title}")
else:
print(f"Multiple matches found: {matches}")
This enhancement empowers users to enter partial artist names, and the program responds with suggestions or displays information if a single match is found. This feature enriches the program's adaptability, addressing common user input scenarios and providing a more intuitive interaction.
Testing and Debugging
Testing and debugging are crucial aspects that contribute to the overall reliability and functionality of any program. Students are advised to conduct thorough testing to validate the accuracy of the functionalities they have implemented and to identify any potential bugs or issues that might arise. Leveraging Python's built-in testing frameworks, such as unit test or pytest, can greatly streamline the testing process, making it more organized and systematic. These frameworks offer a structured approach to testing, allowing for the efficient identification and resolution of any encountered issues. By utilizing these tools, students can ensure that the program operates smoothly and reliably, minimizing the risk of unexpected errors and enhancing the overall user experience. A well-tested and debugged program not only demonstrates the student's proficiency in programming but also instills confidence in the reliability and stability of the software, making it more robust and ready for deployment in real-world applications.
Conclusion
This exploration into creating a Python program for displaying art titles based on artist names serves as a foundational step for students venturing into the intersection of technology and art. Through this guide, they've navigated key aspects of Python programming, delving into data structures, input handling, and string manipulation. The flexibility inherent in the program opens avenues for future enhancements, be it interfacing with external data sources or embracing a graphical user interface. Beyond meeting university assignment criteria, this coding journey empowers students with practical skills applicable across diverse programming contexts, fostering a deeper understanding of the dynamic capabilities of Python in real-world applications.