Writing Python Code to Find Maximum Values in Subsections of a 2D Array is a crucial skill for students across academic disciplines. Python, known for its simplicity and readability, serves as an ideal entry point for beginners venturing into the world of programming. This blog post delves into the intricacies of writing Python code designed to identify maximum values within subsections of a 2D array, offering an invaluable resource to students tackling university assignments or seeking assistance with their Python assignment to augment their Python proficiency. By understanding and implementing this skill, students can strengthen their problem-solving abilities and gain a deeper insight into the fundamental concepts of coding. As Python continues to grow in popularity, mastering it provides a versatile tool for students to apply in data analysis, image processing, and a wide array of academic and professional contexts. With this knowledge in their repertoire, students can better navigate their coursework, improve their coding abilities, and ultimately thrive in an increasingly digital and data-driven world. Whether one is a newcomer to programming or a seasoned coder, the ability to efficiently find maximum values within subsections of a 2D array exemplifies the universal applicability of Python, fostering a stronger foundation in programming and computer science, and enabling students to approach complex problems with confidence and competence.
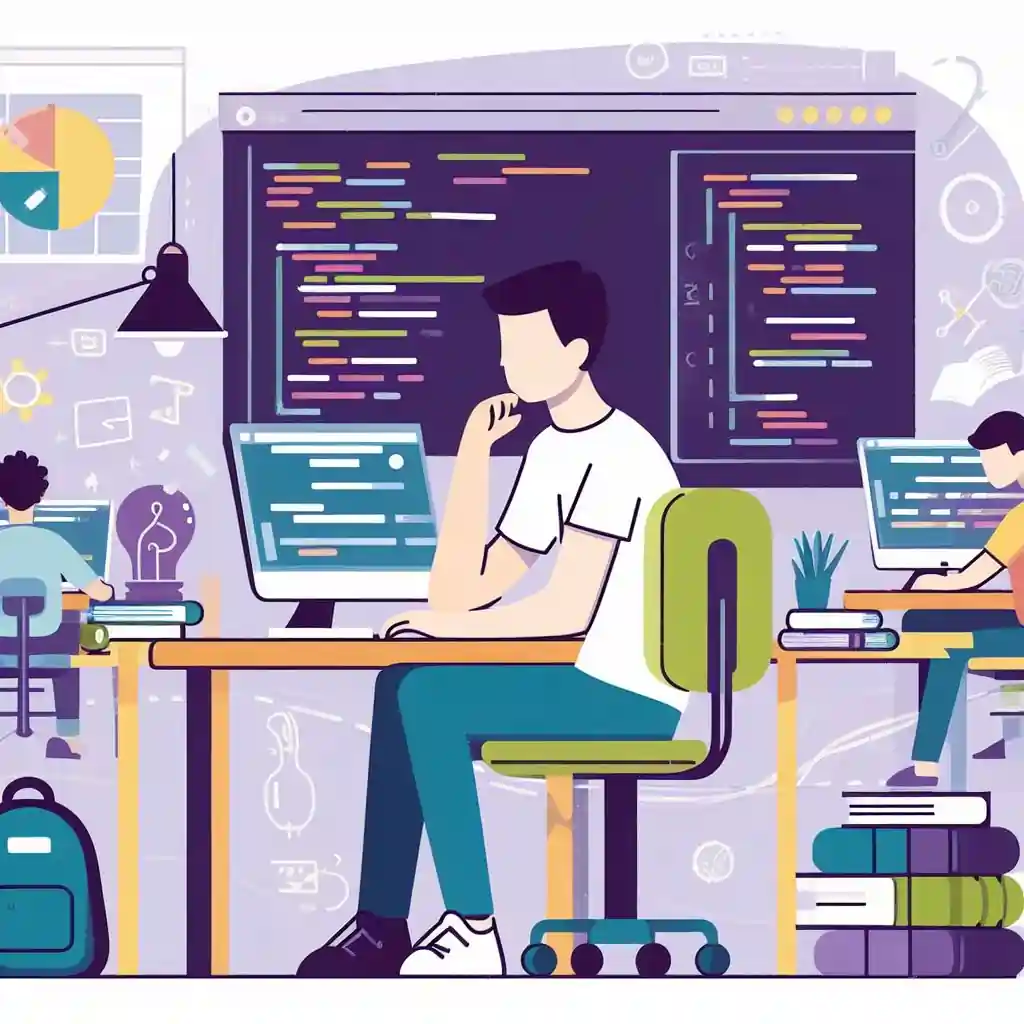
Understanding the Problem
Understanding the problem is a crucial preliminary step before delving into the intricacies of the code. In this context, the challenge at hand revolves around a 2D array, often referred to as a matrix, comprising numerical values as its constituent elements. The primary objective is to pinpoint the maximum value ensconced within specific subsections of this array. In essence, we endeavor to partition the 2D array into smaller, more manageable regions and discern the most substantial element within each of these demarcated sectors. This computational challenge transcends boundaries, finding applications across diverse academic disciplines, including but not limited to computer science, mathematics, and data analysis. Its versatile utility is readily evident in fields such as image processing, data mining, and more, where the ability to isolate and extract the highest values from subsections of data proves invaluable. Whether employed in academia or practical problem-solving scenarios, the pursuit of this solution cultivates a fundamental skill set, underlining the universality of computational techniques and their pervasive relevance in our data-driven world. Thus, the capacity to discern the maximum values within subsections of a 2D array signifies not only a foundational concept but a gateway to more complex problem-solving methodologies, empowering individuals to address a wide spectrum of challenges with precision and computational finesse.
Setting up Python Environment
Setting up a Python environment is the foundational step on your coding journey. Ensuring that you have Python installed on your system is essential. If it's not already on your computer, you can easily obtain it from the official Python website (https://www.python.org/downloads/), where you'll find the latest version ready for download. However, having Python alone is not sufficient; you also require a conducive space for writing, editing, and executing your Python code. This is where a code editor or an integrated development environment (IDE) comes into play. There are several popular choices to pick from, each tailored to cater to different preferences and needs. Visual Studio Code offers a versatile and feature-rich experience, PyCharm is renowned for its Python-specific capabilities and Jupyter Notebook provides an interactive environment well-suited for data analysis and scientific computing. Selecting the right environment largely depends on your workflow and objectives, but regardless of your choice, a well-configured Python environment is the cornerstone for coding success, ensuring that you can seamlessly create, modify, and run Python programs.
Defining the Problem Statement
When confronted with the challenge of analyzing subsections within a 2D array, the primary objective is to pinpoint the maximum value embedded within each specific subsection. These subsections can be precisely demarcated by establishing a defined range of rows and columns within the overarching 2D array. For instance, envisioning a 2D array characterized by 'm' rows and 'n' columns, a subsection is delineated by the indices denoted as (i, j) and (k, l), where 'i' and 'k' signify the initial and terminal rows, while 'j' and 'l' denote the commencement and culmination columns, correspondingly. The crux of the problem revolves around discerning the highest value harbored within the subsection stipulated by these designated indices, thereby necessitating an intricate comprehension of the intricate interplay between rows and columns within the context of the broader 2D array structure.
Approach and Algorithm
In addressing the task of identifying maximum values within subsections of a 2D array, a methodical approach is essential for a systematic solution.
- Input the 2D Array: The initial step involves obtaining the 2D array, a versatile data structure representing various data types like images, matrices, or datasets awaiting analysis.
- Specify Subsections: Define the subsections where the pursuit of maximum values will unfold. This task necessitates establishing precise boundaries and determining the range of rows and columns for each subsection within the array.
- Iterate Through Subsections: Implementation requires the use of nested loops to methodically traverse each subsection of the array. Within each subsection, systematic iteration identifies the maximum value through a comprehensive examination of the data.
- Store Results: Vigilant record-keeping is vital to track the maximum values discovered in each subsection. Utilizing an appropriate data structure, such as a list or an array, facilitates efficient storage and retrieval.
- Output the Results: The final stage involves presenting or utilizing the results as needed. This could encompass displaying the maximum values or seamlessly integrating them into subsequent computational processes. This systematic approach not only streamlines the solution to 2D array challenges but also promotes structured, efficient coding practices in Python.
Creating a 2D Array
Creating a 2D array in Python is a foundational concept, achieved through the use of nested lists. This arrangement allows for the organization of data in a grid-like structure, with rows and columns. For this illustration, consider the following 2D array:
matrix = [
[5, 8, 12, 3],
[14, 6, 9, 2],
[7, 10, 11, 1],
[13, 4, 15, 0]
]
In this example, we have a 4x4 matrix, with each element being a numerical value. Nested lists provide a straightforward means of constructing and manipulating these arrays, as elements can be accessed using row and column indices. This fundamental concept forms the basis for various data manipulation and analysis tasks, making it a valuable skill for students across a range of academic disciplines. Understanding how to create, access, and modify 2D arrays in Python is essential for working with data, solving mathematical problems, or processing information in diverse areas of study, from computer science to engineering and beyond.
Writing the Code
Writing the code to find maximum values in subsections of a 2D array is the practical application of our problem-solving strategy. In this phase, we take our conceptual understanding and translate it into functional Python code. Beginning with a straightforward approach and gradually enhancing it for efficiency is a methodical and pedagogically sound approach. It allows students to grasp the fundamental concepts before delving into more complex optimization techniques.
By starting with simplicity, we build a strong foundation in Python programming and problem-solving, which is invaluable for students in various academic disciplines. As we progress through the code-writing process, we encourage students to think critically, analyze the problem, and come up with solutions that are not only effective but also efficient. This process fosters creativity and logical thinking, which are essential skills in computer science and many other fields. Ultimately, the act of coding and refining the code is where theory transforms into practical knowledge, empowering students to take on real-world challenges and excel in their academic assignments.
Naive Approach
def naive_max_subsections(matrix, sub_size):
max_values = []
for i in range(len(matrix) - sub_size + 1):
for j in range(len(matrix[0]) - sub_size + 1):
max_val = -float('inf')
for x in range(sub_size):
for y in range(sub_size):
max_val = max(max_val, matrix[i + x][j + y])
max_values.append(max_val)
return max_values
In this code, we define a function naive_max_subsections that takes the matrix and the size of the subsections as input. It then iterates through all possible subsections, calculates the maximum value for each subsection, and appends it to the max_values list.
Let's use this function to find the maximum values in 2x2 subsections:
result = naive_max_subsections(matrix, 2)
print(result)
In our quest to optimize the process of finding maximum values within subsections of a 2D array, we embark on a sophisticated approach that leverages the power of dynamic programming. While the naive method serves as a functional starting point, it exhibits limitations when confronted with larger matrices or substantial subsection sizes. To address this challenge, we delve into the realm of dynamic programming, a concept that proves invaluable in the world of algorithm design and optimization.
Dynamic programming, known for its efficiency in solving complex problems, involves breaking down a problem into smaller subproblems and storing their solutions to prevent redundant calculations. In this context, our mission is to precompute the maximum values for all conceivable subsections of the matrix and store these values in a separate 2D array. This precomputation sets the stage for rapid and efficient retrieval of maximum values for any subsection, bypassing the need for redundant calculations.
def preprocess_max_subsections(matrix):
rows = len(matrix)
cols = len(matrix[0])
max_values = [[0] * cols for _ in range(rows)]
for i in range(rows):
for j in range(cols):
max_values[i][j] = matrix[i][j]
for i in range(rows):
for j in range(cols):
if i > 0:
max_values[i][j] = max(max_values[i][j], max_values[i - 1][j])
if j > 0:
max_values[i][j] = max(max_values[i][j], max_values[i][j - 1])
return max_values
def optimized_max_subsections(matrix, max_values, sub_size):
max_subsections = []
rows = len(matrix)
cols = len(matrix[0])
for i in range(rows - sub_size + 1):
for j in range(cols - sub_size + 1):
max_val = matrix[i + sub_size - 1][j + sub_size - 1]
if i > 0:
max_val = max(max_val, max_values[i - 1][j + sub_size - 1])
if j > 0:
max_val = max(max_val, max_values[i + sub_size - 1][j - 1])
max_subsections.append(max_val)
return max_subsections
This optimized approach exemplifies the power of algorithmic thinking, a fundamental skill for students and aspiring programmers. It not only facilitates the solution of immediate problems but also equips learners with a strategic mindset, which can be applied to a myriad of challenges across the academic landscape and professional world. This optimization journey reflects the iterative nature of problem-solving in the realm of computer science and beyond, where efficiency and elegance are paramount.
Here's how you can use the optimized approach to find the maximum values in 2x2 subsections:
max_values = preprocess_max_subsections(matrix)
result = optimized_max_subsections(matrix, max_values, 2)
print(result)
Conclusion
In this blog post, we explored how to write Python code to find maximum values in subsections of a 2D array. We started with a naive approach and then improved it for efficiency using dynamic programming. This knowledge is valuable for students working on university assignments that involve data processing, algorithm design, or coding in Python. Learning how to work with 2D arrays and optimize code for specific tasks is a fundamental skill for programmers. Python's simplicity and readability make it an excellent choice for students to build a strong foundation in coding. Whether you're a beginner or an experienced coder, understanding and implementing efficient algorithms like the one we discussed here can help you tackle a wide range of real-world problems in your academic and professional journey.