Compiler design and construction play a crucial role in the world of computer science and software engineering. Understanding how a compiler works is fundamental for any aspiring programmer, especially for students pursuing computer science degrees. A compiler translates high-level programming languages like C into machine code, enabling computers to understand and execute our code. The focus of this blog post is to delve into the intricacies of fortifying a simple compiler written in the C programming language by incorporating support for arrays and functions. By doing so, the intention is to furnish students with a potent toolset, nurturing their capacity to grapple with university assignments with heightened efficiency and comprehension. The augmentation process involves a sequential evolution of key components within the compiler architecture. Initially, attention is directed towards the lexical analysis phase, wherein the lexer is imbued with the capability to discern array-related syntax, identifying declarations and access operations.
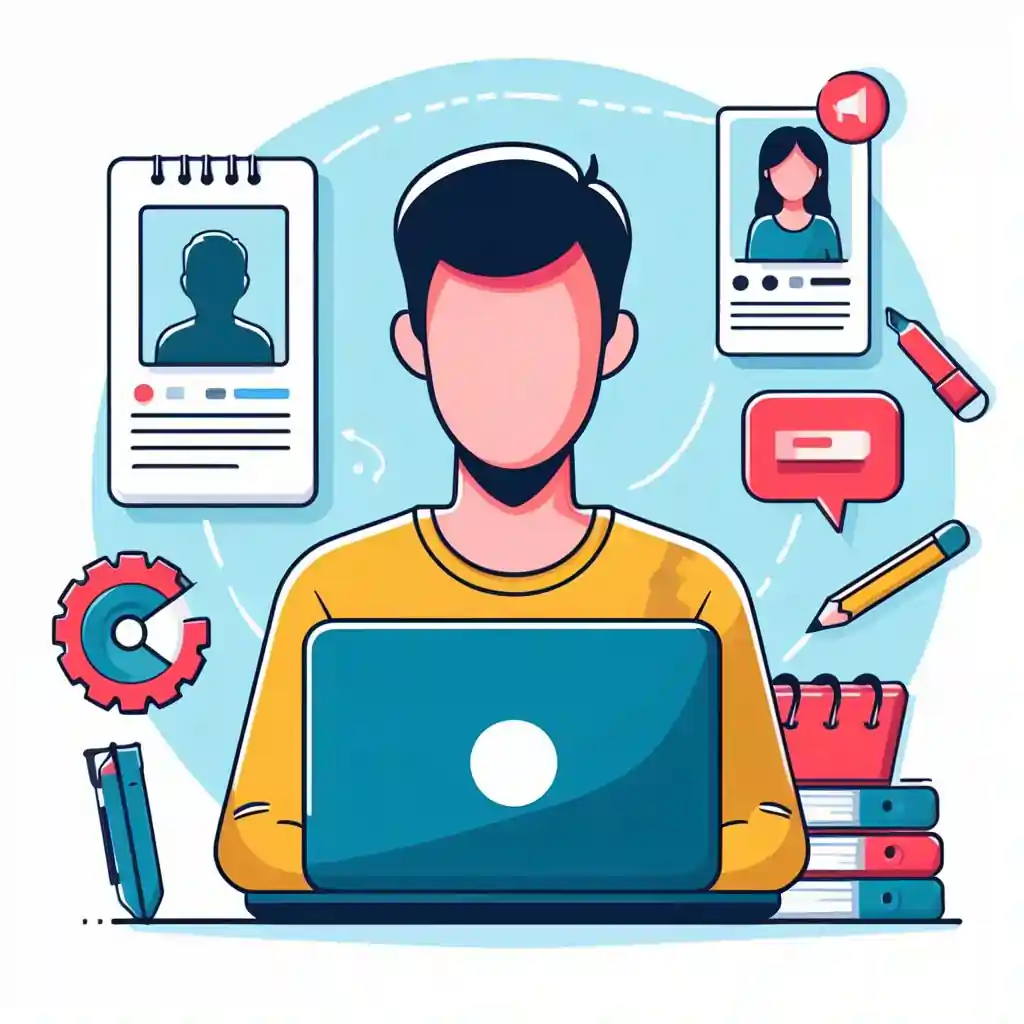
Subsequently, the parser undergoes augmentation to encompass the hierarchical structures of arrays in the syntax tree. Simultaneously, the semantic analyzer is refined to enforce rules governing correct array usage, thereby fortifying the compiler against potential pitfalls. The evolution does not halt at arrays; it extends into the realm of functions. The lexer and parser are extended to recognize function declarations, paving the way for the integration of functions into the compiler's lexicon. Function calls become a focal point, demanding meticulous parsing and semantic analysis to ensure correctness. The code generator, a pivotal component in the compilation process, is then tailored to accommodate the intricacies of array assignments and access, as well as the generation of machine code for function calls and returns. Beyond these technical enhancements, the blog advocates for robust error handling and debugging features, emphasizing their pivotal role in the educational journey. A compiler with informative error messages and debugging capabilities serves as a didactic tool, guiding students through the nuances of identifying and rectifying issues in their source code. The imperative of comprehensive documentation, elucidating the implementation details of arrays and functions within the compiler, is underscored. This documentation, accompanied by illustrative examples, serves as a compass, guiding students through the augmented compiler's terrain. In essence, this comprehensive evolution of a simple compiler in C acts not merely as a technical tutorial but as a pedagogical instrument, fostering a symbiotic relationship between students and the complexities of compiler construction, thereby paving the way for a more enriched and empowered cohort of programming aficionados seeking assistance with their C assignment.
The Foundation: Understanding the Simple Compiler
Establishing a solid foundation is imperative before delving into the intricacies of enhancing a simple compiler. It's essential to grasp the fundamental structure and functionality inherent to these systems. A basic compiler, as a cohesive entity, comprises integral components that collectively bring about the transformation of high-level programming instructions into executable machine code. These cardinal components include a lexer, responsible for analyzing the source code and segmenting it into distinct tokens; a parser, tasked with constructing a hierarchical syntax tree that represents the syntactic structure of the code; a semantic analyzer, pivotal for scrutinizing adherence to language-specific rules and ensuring the correctness of the code's semantics; a code generator, which translates the syntax tree into machine code comprehensible to the target architecture; and finally, an optimizer, a crucial component refining the generated code to enhance its efficiency and performance. This systematic breakdown elucidates the orchestrated dance of these components, each playing a distinct yet interconnected role in the intricate symphony of compiler functionality. The lexer initiates the process by tokenizing the source code, setting the stage for the parser to weave a syntactic tapestry. The semantic analyzer acts as a vigilant guardian, ensuring adherence to language rules and semantic accuracy. Subsequently, the code generator undertakes the transformational task, translating the abstract syntax into machine-executable instructions, while the optimizer refines this translation for optimal performance. Understanding this foundational framework lays the groundwork for comprehending the subsequent enhancements in the evolution of the compiler.
Why Add Arrays and Functions?
Before diving into the technical details, let's briefly discuss why adding support for arrays and functions is a significant step in enhancing your compiler.
- Arrays: Arrays are fundamental data structures in C and many other programming languages. They allow you to store and manipulate collections of data. Learning to work with arrays in a compiler will help you understand how to allocate and manage memory efficiently, which is a crucial skill in systems programming. In the realm of compiler development, dealing with arrays enhances your understanding of memory allocation and indexing mechanisms, a core element of translating high-level code into efficient machine instructions.
- Functions: Functions are the building blocks of modular and reusable code. By incorporating function support into your compiler, you'll be enabling students to write more organized and maintainable code. This feature is particularly useful when working on larger projects or designing libraries. Functions enable code modularity, making it easier to develop, debug, and maintain software. A compiler's ability to handle functions correctly is crucial for producing executable code that reflects the structure of the source program accurately.
- Real-World Relevance: Many programming assignments and real-world projects require the use of arrays and functions. By adding support for these features, you are preparing students for real-world challenges and making your compiler more practical. Students who learn to work with arrays and functions in a compiler context gain skills that directly translate to practical software development tasks. Whether they're working on algorithmic problem-solving, system software, or application development, these skills are invaluable.
Now, let's get into the nitty-gritty of how to add arrays and functions to your simple C compiler.
Step 1: Adding Support for Arrays
Lexical Analysis for Arrays:
- The lexer must recognize array declarations and access operations.
- Update the lexer to identify array-related keywords like int[] or float[] and tokenize them accordingly.
Parsing Arrays:
- Expand the parser to handle array declarations and access expressions in the syntax tree.
- Ensure the semantic analyzer checks for correct array usage, preventing index out-of-bounds errors.
Code Generation for Arrays:
- Modify the code generator when generating code to handle array assignments and access.
- This involves calculating memory addresses based on indices and appropriately storing and retrieving array elements.
In the initial step of enhancing the compiler to support arrays, the focus is on augmenting the lexical analysis, parsing, and code generation processes. The lexer is empowered to recognize array-specific syntax, the parser adapts to the hierarchical structures of arrays, and the code generator is refined to seamlessly handle array assignments and access. This systematic evolution lays the groundwork for a compiler capable of interpreting and executing array-related operations with precision and efficiency.
Step 2 Integrating Functions
Function Declarations:
- Extend both the lexer and parser to recognize function declarations.
- Identify tokens like "function" followed by the function name and parameter list.
- Ensure the syntax tree mirrors the hierarchical structure of functions.
Function Calls:
- Update the parser to adeptly handle function calls within the code.
- Enforce semantic analysis to verify the correctness of function calls.
- This involves checking for matching parameter types and the existence of the called function.
Code Generation for Functions:
- Modify the code generator to produce machine code specifically for function calls and returns.
- Implement a mechanism to manage the function call stack effectively.
- This includes the crucial tasks of saving and restoring local variables and control flow during function execution.
In the second phase of compiler enhancement, the focus is on seamlessly integrating functions into the compiler's lexicon. This involves extending both the lexer and parser to recognize function-related syntax and ensuring the syntax tree accurately reflects the hierarchical nature of functions. Function calls are then addressed with updates to the parser and the addition of semantic analysis checks, ensuring the correctness of these calls. The final step involves adapting the code generator to handle the intricacies of generating machine code for functions, including managing the function call stack for proper execution and control flow. This holistic approach transforms the basic compiler into a versatile tool capable of accommodating the complexities of function-oriented programming.
Step 3: Error Handling and Debugging
In the crucial step of enhancing the compiler's robustness, a paramount focus is directed toward fortifying its error handling and debugging capabilities. Robust error-handling mechanisms are integrated to empower the compiler in identifying and addressing issues within the source code comprehensively. This involves the implementation of informative error messages that serve as guiding beacons for students, facilitating the identification and rectification of potential pitfalls. The compiler is further enriched with sophisticated debugging features, adding a layer of diagnostic prowess to the development process. Incorporating line numbers in error messages enhances precision, pinpointing the exact location of errors within the code. Additionally, the compiler gains the ability to generate debug symbols for the compiled code, offering invaluable insights into the program's execution and aiding developers in dissecting complex issues during the debugging phase. This comprehensive approach to error handling and debugging transforms the compiler into a pedagogical tool, not only advancing its technical capabilities but also nurturing a more intuitive and supportive environment for students grappling with the intricacies of programming and compiler construction.
Step 4: Documentation and Examples
In the pivotal phase of advancing the compiler, a strategic emphasis is placed on documentation and illustrative examples, fostering an environment conducive to good programming practices. Comprehensive documentation for the enhanced compiler serves as a cornerstone, offering students a detailed roadmap to navigate through the intricacies of arrays and functions. The documentation meticulously outlines how these features are implemented, elucidating the underlying processes and structures. It goes beyond the mere technicalities by addressing any limitations or special considerations, providing a nuanced understanding that equips students to make informed decisions in their coding endeavors. Crucially, the inclusion of examples and sample programs becomes instrumental in translating theoretical knowledge into practical application. These examples serve as concrete demonstrations, showcasing the correct usage of arrays and functions within the compiler. By presenting tangible instances of successful implementation, students are not only empowered to comprehend these concepts but are also inspired to adopt best practices in their coding practices, thereby elevating the overall standard of their programming acumen. This holistic approach to documentation and examples establishes a foundation for students to not only master the enhanced compiler but also to cultivate a mindset grounded in excellence and precision in their programming pursuits.
A Sample Code Example
Let's look at a simple code example that demonstrates how arrays and functions work together in C:
int sum(int arr[], int size) {
int result = 0;
for (int i = 0; i < size; i++) {
result += arr[i];
}
return result;
}
int main() {
int numbers[5] = {1, 2, 3, 4, 5};
int total = sum(numbers, 5);
return 0;
}
In this code snippet, we have an array number and a function sum. The sum function takes an array and its size as arguments calculates the sum of the elements, and returns the result. In the main function, we declare an array of numbers, populate it with values, and call the sum function with this array.
By adding support for arrays and functions in your compiler, you enable students to compile and run such code, deepening their understanding of how these fundamental features work at a low level.
Challenges and Considerations
Adding arrays and functions to a simple C compiler is a significant step in the right direction, but it comes with its challenges:
- Memory Management: You need to manage memory efficiently, especially when working with arrays. Ensure that you allocate and deallocate memory properly to prevent memory leaks and buffer overflows.
- Error Handling: Implementing robust error handling and reporting mechanisms is crucial. Provide meaningful error messages to help students debug their code effectively.
- Optimization: Consider implementing basic optimizations to produce efficient code. This includes eliminating redundant computations and minimizing the use of registers.
- Testing: Comprehensive testing is essential to ensure that the added features work correctly. Create a suite of test cases that cover various aspects of arrays and functions to validate your compiler's correctness.
Conclusion
Adding support for arrays and functions to your simple C compiler is a commendable endeavor that will greatly benefit computer science students. It provides them with valuable insights into how compilers process essential language features and equips them with skills and knowledge that are directly applicable to their future software development endeavors. By following the steps outlined in this guide and addressing the associated challenges, you can enhance your compiler's capabilities and empower your students to tackle more advanced assignments and projects with confidence. In the process, you'll be fostering a deeper appreciation for the intricacies of compiler construction and the power of understanding how programming languages are translated into machine code.