Delving into the realm of protocol creation with Python unveils an intriguing mix of structured communication standards combined with Python's versatility. Communication protocols dictate how data is formatted, transmitted, and processed between systems, ensuring consistency and reliability. Python emerges as an ideal tool to complete your python assignment due to its simplicity and a rich array of libraries like socket and asyncio, which facilitate both the design and simulation of these protocols. These libraries streamline the establishment of connections, data transmission, and even multi-threaded communication processes. For budding developers or students, understanding Python's role in protocol creation offers not just knowledge but a hands-on ability to simulate real-world communication scenarios, thus bridging theoretical understanding with practical application.
Introduction to Protocol Creation in Python
Delving into the realm of protocol creation with Python unveils an intriguing mix of structured communication standards combined with Python's versatility. Communication protocols dictate how data is formatted, transmitted, and processed between systems, ensuring consistency and reliability.
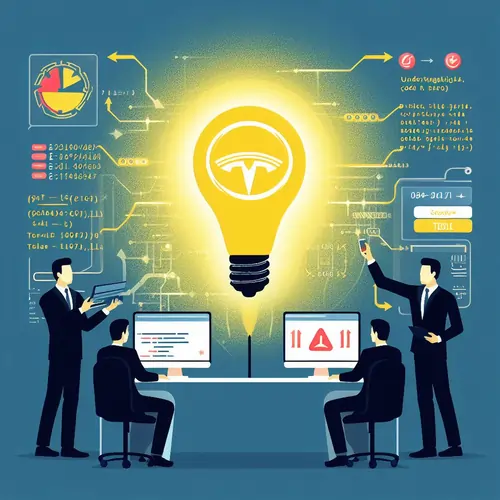
Python emerges as an ideal tool for this due to its simplicity and a rich array of libraries like socket and asyncio, which facilitate both the design and simulation of these protocols. These libraries streamline the establishment of connections, data transmission, and even multi-threaded communication processes. For budding developers or students, understanding Python's role in protocol creation offers not just knowledge but a hands-on ability to simulate real-world communication scenarios, thus bridging theoretical understanding with practical application.
Understanding Protocols
Protocols are crucial in enabling effective communication between different computing systems. They set predefined rules and standards that each data packet must conform to, allowing information to be transferred seamlessly between systems. In Python, we can simulate protocol communication using sockets and other libraries to establish connections, send, and receive data.
Python and Its Application in Communication
Python, with its extensive libraries and versatile functionality, is a preferred language to implement and simulate communication protocols. Libraries like socket and asyncio allow developers to handle both the client and server sides of protocol communication, making Python a suitable choice for learning and developing communication protocols.
Establishing Basic Communication
Establishing basic communication forms the bedrock of creating advanced, specialized communication protocols. Python’s socket library plays an indispensable role in this, enabling developers to create reliable client-server connections. In essence, the server creates a socket, binds it to a specific address and port, and starts listening for incoming connections. On the other hand, a client creates a socket to connect to the server's address. Once the connection is established, data can be sent and received between them. This foundational knowledge in establishing basic communication is crucial for students and aspiring developers as it lays the groundwork for understanding more complex networking concepts, data transmissions, and eventually, the development of sophisticated, secure, and efficient communication protocols.
Using Socket Library
Python’s socket library is a fundamental tool for establishing client-server communication, allowing for the creation of server sockets that can listen for incoming connections and client sockets that can interact with the server. In the server's simplistic example, after binding to a local address and a port, it listens for connections from clients. When a connection is established, it can receive data from the client and send data back. This basic example is pivotal for beginners as it illustrates the foundational concepts behind network programming with Python, providing a stepping stone for developing more complex, robust, and secure network applications and understanding the intricacies of various communication protocols. Below is a simplistic example of a server creating a socket, binding to an address, and listening for incoming connections:
import socket
server_socket = socket.socket(socket.AF_INET, socket.SOCK_STREAM)
server_socket.bind(('localhost', 12345))
server_socket.listen(5)
while True:
client_socket, addr = server_socket.accept()
print(f"Connection from {addr}")
client_socket.send(b'Hello, client!')
client_socket.close()
Creating a Client
The creation of a client using Python's socket library is a relatively straightforward process, providing a clear and concise way to establish a connection to a server. The client initializes a socket and utilizes it to connect to the specified address of the server. This crucial interaction is what facilitates the exchange of data between client and server. Understanding the process of creating a client socket is vital for developers and students aiming to delve deeper into network programming, as it forms the basis of client-server interaction, allowing them to explore more advanced topics like data serialization, encryption, error handling, and protocol development, ultimately enriching their proficiency in developing networked applications with Python. A client needs to create a socket and then connect to the server’s address:
import socket
client_socket = socket.socket(socket.AF_INET, socket.SOCK_STREAM)
client_socket.connect(('localhost', 12345))
data = client_socket.recv(1024)
print(f"Received: {data.decode()}")
client_socket.close()
Simulating a Hypothetical Tesla Protocol
Defining the Protocol
Let’s hypothesize that the Tesla communication protocol involves a secure and efficient exchange of data related to vehicle status, battery levels, and other relevant information. It might have specific message formats, encryption methods, and error-checking mechanisms to ensure data integrity and security.
# Example of a hypothetical message format
class TeslaMessage:
def __init__(self, vehicle_id, battery_level, status):
self.vehicle_id = vehicle_id
self.battery_level = battery_level
self.status = status
Implementing Protocol Logic
Implementing the logic for the hypothetical Tesla protocol involves encoding and decoding messages, handling errors, and ensuring security. For illustrative purposes, let's consider a simplistic approach where messages are serialized using JSON, with basic error checking:
import json
import socket
def send_tesla_message(socket, message):
serialized_message = json.dumps(message.__dict__)
socket.send(serialized_message.encode())
def receive_tesla_message(socket):
data = socket.recv(1024).decode()
message_dict = json.loads(data)
return TeslaMessage(**message_dict)
Enhancing Protocol Security and Efficiency
Enhancing Protocol Security and Efficiency within the realm of Python involves deploying a myriad of strategies and techniques to fortify data transmission against security vulnerabilities and to optimize the performance of the communication process. Employing Python's extensive libraries, developers can integrate encryption methods to secure data, thus safeguarding information integrity. Additionally, implementing error-checking mechanisms can help in early detection and correction of data transmission inaccuracies, promoting reliability in communication. Optimizing protocol efficiency and security is paramount in developing robust applications, ensuring the swift and secure exchange of information. These enhancements are crucial learning components for students and developers, providing them with the skills to create advanced, resilient, and efficient communication protocols in Python.
Adding Encryption
In the digital age, the significance of security in communication protocols cannot be overstated. With the ever-present threat of data breaches and cyberattacks, encryption has become a linchpin in safeguarding data transmissions. By converting plain text into an unreadable format, encryption ensures that intercepted messages remain indecipherable to unauthorized entities. Python, renowned for its versatility, offers the cryptography library, a powerful tool that simplifies the implementation of encryption and decryption mechanisms. With this library, developers can seamlessly integrate high-level cryptographic operations into their applications, providing a robust shield against potential threats. For aspiring developers and students, mastering Python's encryption tools is essential, laying the foundation for building secure and trustworthy communication systems. Python's cryptography library can be used to implement encryption and decryption:
from cryptography.fernet import Fernet
key = Fernet.generate_key() # This key must be securely shared between client and server
cipher_suite = Fernet(key)
def encrypt_message(message):
return cipher_suite.encrypt(message.encode())
def decrypt_message(encrypted_message):
return cipher_suite.decrypt(encrypted_message).decode()
Implementing Error Checking
Error checking is crucial in maintaining the integrity of communications within any protocol, acting as a sentinel against the loss or corruption of data during transmission. A widely adopted method is the use of checksums—a computed value that represents the data and is sent along with each message. This checksum is then recalculated at the receiving end and compared to the original; any discrepancy signals an error in transmission, prompting corrective action. This method is relatively simple to implement but profoundly impacts the reliability of data communication, ensuring the received data is a true representation of the original, thus fostering trust and accuracy in communication systems. In essence, understanding and implementing error-checking mechanisms are pivotal steps in mastering robust and reliable network programming. A simple method is to include a checksum with each message:
import hashlib
def generate_checksum(message):
return hashlib.md5(message.encode()).hexdigest()
def verify_checksum(message, checksum):
return hashlib.md5(message.encode()).hexdigest() == checksum
Putting it All Together
Now, we can use these building blocks to create a hypothetical Tesla communication protocol, where messages are securely sent and received with error checking:
import socket
import json
from cryptography.fernet import Fernet
# Initialize encryption
key = Fernet.generate_key()
cipher_suite = Fernet(key)
# Initialize server socket
server_socket = socket.socket(socket.AF_INET, socket.SOCK_STREAM)
server_socket.bind(('localhost', 12345))
server_socket.listen(5)
while True:
client_socket, addr = server_socket.accept()
print(f"Connection from {addr}")
# Receive and decrypt message
encrypted_message = client_socket.recv(1024)
message = decrypt_message(encrypted_message)
# Deserialize and verify checksum
message_dict, checksum = json.loads(message)
received_message = TeslaMessage(**message_dict)
if not verify_checksum(json.dumps(received_message.__dict__), checksum):
print("Error: Checksum mismatch")
else:
print(f"Received valid message: {received_message.__dict__}")
# Send a response back
response_message = TeslaMessage('Server', '100%', 'OK')
serialized_response = json.dumps({
'message': response_message.__dict__,
'checksum': generate_checksum(json.dumps(response_message.__dict__))
})
encrypted_response = encrypt_message(serialized_response)
client_socket.send(encrypted_response)
client_socket.close()
Conclusion
While the "Tesla communication protocol" is purely hypothetical, Python’s extensive libraries and versatility make it an ideal language for learning, simulating, and developing communication protocols. Through this conceptual exploration, students can understand the fundamental components of communication protocols, including message formatting, encoding/decoding, security through encryption, and error checking. Developing a deeper comprehension of these aspects can significantly aid students in solving Python assignments related to communication protocols and network programming.