Creating a Java program with a text-based UI for hotel room bookings is a valuable endeavor for university students. It presents an opportunity to bridge the gap between theoretical knowledge and practical application, a challenge frequently encountered in academic settings. University assignments often demand the application of programming skills to real-world scenarios, and this project provides precisely that. It offers a practical avenue for students to develop, refine, and demonstrate their Java programming capabilities, ultimately helping them complete their Java assignment. This blog aims to be a comprehensive guide through the intricate process of designing and implementing a Java program for hotel room bookings. The steps involved are broken down into manageable components, ensuring that even students with varying levels of programming experience can embark on this journey. Whether the project is a rudimentary room reservation system or an intricate application featuring advanced functionalities such as room availability checks and payment processing, it remains adaptable to suit diverse skill sets.
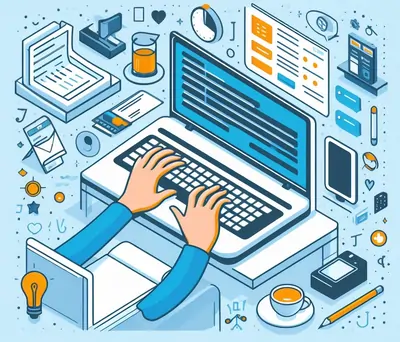
One of the project's merits is its applicability to a wide array of academic disciplines. Be it a Java programming course, software engineering class, or database management study, this project can serve as an excellent submission for assignments. The universality of the hotel booking system concept is advantageous, as students can adapt it to different course requirements and project scopes, allowing them to tailor the project to their specific needs.
Furthermore, the collaborative aspect of this project shouldn't be overlooked. It provides an opportunity for students to work individually or in teams, fostering collaboration and teamwork, skills that are highly valued in the professional world. These collaboration experiences enrich students' problem-solving skills and expose them to different perspectives, thereby enhancing their overall learning experience.
Why Create a Hotel Room Booking System?
Before we dive into the technical aspects of creating a Java program for hotel room bookings, let's discuss the reasons why such a system can be beneficial for university students.
- Real-World Relevance: Hotel room booking systems are widely used in the hospitality industry. Building a simplified version of this system allows students to work on a project that has real-world relevance. This experience will prepare them for similar scenarios in their future careers and help them understand how technology supports the daily operations of businesses.
- Complexity: This project can be as simple or as complex as needed, making it suitable for students at various skill levels. It can be a basic reservation system or a more advanced one with features like room availability checks and payment processing. This adaptability ensures that students can tailor the project to their skill level and the course requirements.
- Hands-On Experience: Students can gain practical experience in various aspects of software development, including user interface design, data handling, and error handling. This hands-on experience is invaluable for reinforcing the theoretical knowledge gained in lectures and textbooks. It allows students to see how concepts are applied in real-world projects.
- Collaboration: Students can work on this project individually or in teams, promoting collaboration and teamwork. In the professional world, collaborative skills are highly prized, and this project provides an opportunity for students to practice them. Working in teams allows students to learn from each other, share ideas, and divide tasks efficiently.
- Applicability: This project can be used for assignment submissions in courses related to Java programming, software engineering, or database management. Its versatility makes it applicable in various educational contexts, from introductory programming courses to advanced software engineering classes. Professors can adjust the project's complexity to align with the course's learning objectives, ensuring its applicability in different academic settings.
Technical Implementation
To embark on the journey of creating a Java program with a text-based user interface (UI) for hotel room bookings, it's imperative to disassemble the process into discrete components. This meticulous approach ensures that each aspect of the program is well-considered and expertly implemented.
- Data Model: The cornerstone of our hotel room booking program is the data model. Here, we grapple with the task of representing the hotel and its myriad rooms. This necessitates the utilization of classes and data structures to meticulously craft a blueprint of the information. Each room should be imbued with a unique set of attributes, including its room number, availability status, price, and occupancy details. This detailed data model forms the bedrock upon which the entire program is constructed.
- User Interface: Given that our program adopts a text-based approach, the user interface (UI) is decidedly sans graphical embellishments. Instead, the UI comprises an assemblage of text menus and prompts. Java's versatile Scanner class assumes the pivotal role of a communication bridge, facilitating user input acquisition and information display. The art lies in designing an intuitive and user-friendly interface that guides users through the booking process seamlessly.
- Booking Logic: Beneath the surface, the program must house a robust booking logic that orchestrates the intricate dance of room availability checks, reservation creation, and cost calculations. To accomplish this, algorithmic prowess is a must. Data structures like arrays or lists emerge as indispensable tools for managing bookings and monitoring room availability. The intricacies of this logic determine the program's functionality and reliability.
- Error Handling: In the real world, users are prone to err, and our program should be primed to handle such inevitable mishaps gracefully. Vigilant error handling mechanisms must be interwoven into the codebase. These mechanisms are designed to anticipate scenarios where users input erroneous data or endeavor to book already occupied rooms. The program's resilience is gauged by its ability to provide clear and informative responses in such situations.
- File I/O (optional): Elevating the program's robustness to the next level is the optional incorporation of File Input/Output (I/O) functionality. This feature endows the program with the capability to persistently store and retrieve booking data from a file. Even when the program is closed and subsequently reopened, bookings remain intact. This enhances the program's utility and user experience.
- Testing: The crucible in which the program's mettle is tested is the rigorous testing phase. Each facet of the program must be scrutinized to ensure it functions as envisioned. This includes not only the typical use cases but also the edge cases. Rigorous testing encompasses scenarios such as fully booking the hotel, canceling reservations, and gracefully handling erroneous user inputs. The effectiveness of the program hinges on the thoroughness of its testing regime.
- Documentation: Last but certainly not least is the imperative to document the codebase comprehensively. This entails the judicious inclusion of comments and explanatory notes throughout the code. Such documentation serves a dual purpose: aiding the programmer's own understanding of the code and providing clarity to potential code reviewers. In the realm of collaborative software development, meticulous documentation is the glue that holds the project together.
Getting Started with the Java Program
Now, let's embark on our journey to create a Java program for hotel room bookings. This endeavor begins with breaking down the project into manageable steps, providing a structured approach that simplifies the process for students and aspiring developers. By segmenting the project into smaller components, we facilitate a gradual understanding and implementation of the various features, making it accessible to individuals with varying levels of programming experience. The step-by-step approach ensures that even those new to Java can follow along and steadily build a functional hotel room booking system. These initial stages of the project, from setting up the programming environment to designing the text-based user interface, lay the foundation for the subsequent development work. Through this methodical process, students can gain confidence and gradually build their expertise in Java programming, while creating a practical, real-world solution that serves as a testament to their growth and capabilities.
Step 1: Setting Up the Project
Begin by establishing a new Java project within your preferred integrated development environment (IDE) like Eclipse, IntelliJ, or NetBeans. This initial stage provides the essential groundwork for your hotel room booking system. Configuring the project within the IDE allows for efficient code management and project structuring. Once this foundational step is completed, you can seamlessly initiate the creation of the program's main Java class. This class serves as the core of your application, housing the logic and functionalities necessary for managing hotel room reservations. A well-structured and organized project environment is pivotal for the development process, ensuring clarity, ease of debugging, and efficient coding as you proceed with building the application.
Step 2: Designing the Text-Based UI
Designing the user interface is a crucial part of this project. Even though it's text-based, it should be user-friendly and easy to navigate. Consider using a simple menu-driven approach. Here's a basic example:
public class HotelBookingSystem {
public static void main(String[] args) {
while (true) {
System.out.println("Welcome to the Hotel Booking System");
System.out.println("1. View available rooms");
System.out.println("2. Make a reservation");
System.out.println("3. Cancel a reservation");
System.out.println("4. Exit");
// Read user input and perform corresponding actions
int choice = getUserChoice();
switch (choice) {
case 1:
// View available rooms
break;
case 2:
// Make a reservation
break;
case 3:
// Cancel a reservation
break;
case 4:
System.out.println("Goodbye!");
System.exit(0);
break;
default:
System.out.println("Invalid choice. Please try again.");
}
}
}
// Helper method to get user input
private static int getUserChoice() {
// Implement input validation here
return 0; // Replace with actual user input
}
}
Step 3: Managing Hotel Room Data
For a hotel room booking system, you'll need to manage data related to rooms, reservations, and availability. Here are some key considerations:
Room Data
You can create a class to represent individual hotel rooms. This class should contain information such as room number, room type (e.g., single, double, suite), and price per night.
public class Room {
private int roomNumber;
private String roomType;
private double pricePerNight;
// Constructors, getters, and setters
}
Reservation Data
Create a class to represent reservations, including the guest's name, check-in and check-out dates, and the room they've booked.
public class Reservation {
private String guestName;
private Date checkInDate;
private Date checkOutDate;
private Room bookedRoom;
// Constructors, getters, and setters
}
Managing Availability
To track room availability, you can maintain a list of rooms and reservations. Whenever a reservation is made, you'll need to update room availability accordingly.
Step 4: Implementing Business Logic
The core of your program lies in implementing the business logic. Here are some examples of what you might need to do:
- Viewing available rooms: Iterate through the list of rooms and display only those that are not reserved for the selected date range.
- Making a reservation: Allow users to enter their details, choose a room, and confirm the reservation. Ensure that the room is available for the selected dates.
- Canceling a reservation: Allow users to enter their reservation details and remove the reservation from the list.
Step 5: Error Handling
Handling errors and edge cases is an indispensable aspect of software development. Robust error handling is vital to ensure that the program responds gracefully to unexpected situations. In the context of a hotel room booking system, you should implement error handling mechanisms to address various scenarios. For instance, the system should detect and respond to invalid input, such as entering dates that are out of range or attempting to reserve a room that doesn't exist. Additionally, it should account for situations where users attempt to book a room that is already reserved, ensuring that such cases are handled gracefully without causing system crashes or data corruption. Effective error handling enhances the system's reliability and user experience.
Step 6: Data Persistence
Implementing data persistence is often a critical requirement in software applications. For a hotel room booking system, it involves storing reservation data and room information for future access. This data can be saved using file input/output (I/O) operations, where reservations are written to and read from files, offering a basic form of data storage. However, for more advanced projects or those with extensive data management needs, integrating a database becomes a suitable solution. Database management systems, such as MySQL, PostgreSQL, or SQLite, allow for efficient storage, retrieval, and querying of data, providing scalability and reliability. The choice between file I/O and a database largely depends on the project's complexity and requirements. Integrating data persistence ensures that reservation records and room details are maintained even after the program is closed, making it a vital component of a comprehensive hotel room booking system.
Testing and Debugging
In the critical phases of testing and debugging, a meticulous approach is indispensable before deeming the project as complete. The imperative task is to rigorously test every functionality integrated into the program, meticulously scrutinizing its behavior to guarantee that it aligns with the anticipated outcomes. Thorough testing not only ensures the reliability of the program but also enhances its overall performance. Leveraging the debugging tools inherent in your Integrated Development Environment (IDE) becomes invaluable during this stage. These tools empower developers to identify and rectify any anomalies or errors that might impede the program's seamless execution. Addressing these issues systematically not only contributes to a more robust and error-resistant program but also cultivates a skill set crucial for real-world software development scenarios. Consequently, instilling a comprehensive testing and debugging regimen in the development process is not just a procedural necessity but a fundamental stride towards producing software that stands resilient against potential glitches and discrepancies.
Conclusion
In conclusion, embarking on the journey of creating a Java program with a text-based UI for hotel room bookings offers a myriad of benefits to university students and aspiring developers. This project not only bridges the gap between theoretical knowledge and practical application but also provides a platform to nurture creativity and problem-solving skills. As students navigate through the various stages, from project setup to data persistence, they gain a holistic understanding of software development. It prepares them for the real-world challenges they will encounter in their careers, equipping them with hands-on experience, teamwork, and error-handling skills. Whether used as an assignment in Java programming, software engineering, or database management courses, this endeavor is an educational asset that encourages students to think critically, innovate, and apply their knowledge, ensuring they are well-prepared for the dynamic world of software development.