Master's students pursuing their degrees in universities often face complex assignments that require them to apply various algorithms and data structures. One common problem involves navigating and exploring maps, a task that can be significantly simplified through the use of directed networks. In this blog, we will explore how simulating map exploration using directed networks can complete your C assignment and assist master's students in solving assignments, with a specific focus on implementing this approach in the C programming language. Before delving into the solution, let's establish a clear understanding of the problem. Assignments in computer science often involve tasks related to graph theory and algorithms, and map exploration can be framed as a graph problem. In this context, the map is represented as a graph, where locations are nodes, and roads or paths between locations are edges. Each edge is directed to indicate one-way streets or paths, and students are tasked with finding routes, calculating distances, or determining optimal paths between locations. Directed networks, also known as directed graphs, are particularly well-suited for modeling map exploration scenarios. Here are some benefits of using directed networks in this context: Realistic Representation: Directed edges allow you to model one-way streets and directional restrictions accurately, reflecting real-world road networks. Efficient Pathfinding: Directed graphs enable efficient algorithms for pathfinding, such as Dijkstra's algorithm and A* search, which are essential for solving navigation problems.
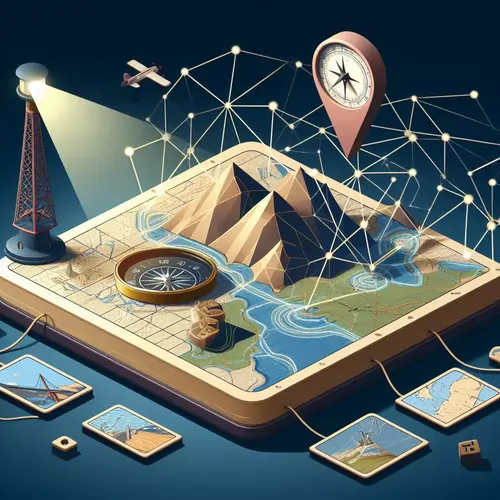
Flexibility: Directed networks can be adapted to represent various types of maps, from city streets to campus layouts, making them versatile for different assignment scenarios. Implementing directed networks in C involves several steps. First, you define a struct for nodes and edges, allowing you to represent locations and directed roads accurately. Then, you create data structures for graph representation, using arrays or linked lists for nodes and edges, adjusting the maximum values based on the assignment's requirements. Afterward, you build the directed graph by populating it with nodes and edges to simulate the map. Students can gain valuable experience by implementing pathfinding algorithms in C. Dijkstra's algorithm and A* search are commonly used for finding the shortest paths in directed graphs. By providing students with these tools, you help them enhance their problem-solving skills and prepare for more complex challenges in their academic and professional careers. For a more interactive experience, you can develop a user interface using C's graphics libraries or platforms like SDL, allowing students to input starting and ending locations, visualize the map, and view the computed paths. Simulating map exploration using directed networks in C not only simplifies complex assignments but also equips master's students with practical skills that are valuable in both academic and professional settings. Whether it's navigating city streets or campus pathways, mastering directed networks in C will undoubtedly be a valuable skill for any computer science student, and the techniques outlined in this blog can serve as a solid foundation for tackling a wide range of assignment scenarios and real-world applications.
Understanding the Basics:
Before we start coding, it's crucial to have a clear understanding of the problem statement. In this assignment, we are tasked with simulating the exploration of a map using a directed network. The map consists of nodes (locations) and directed edges (paths) connecting these nodes. Our goal is to write a C program that allows us to explore this map, finding paths from a starting location to a destination location.
- Directed Network (Graph): In this context, a directed graph forms the basis for simulating the exploration of a map. Visualize it as a map where nodes represent locations, and edges signify the paths between them. The crucial aspect is that the direction of the edges indicates the one-way nature of the paths, mimicking the reality of navigating through different locations.
- Adjacency Matrix: Before delving into the C code, it's essential to grasp the concept of an adjacency matrix. This 2D array serves as a representation of the connections between nodes in the directed graph. For a directed graph, the adjacency matrix provides a clear indication of whether there is an edge from one node to another, laying the groundwork for defining the structure of the map.
- Depth-First Search (DFS): As part of the foundational understanding, the Depth-First Search (DFS) algorithm comes into play. DFS is a traversal algorithm that operates by exploring as far as possible along each branch before backtracking. In the context of map exploration, DFS becomes a powerful tool to simulate paths, allowing for a systematic exploration of locations. The algorithm marks visited nodes, ensuring comprehensive coverage of the map.
Benefits of Using Directed Networks
Directed networks, also known as directed graphs, offer numerous advantages when it comes to modeling map exploration scenarios for the benefit of master's students studying in universities, particularly when utilizing the C programming language. Here, we outline the benefits of using directed networks in this context:
- Realistic Representation: Directed networks allow for a realistic representation of map exploration scenarios by accurately modeling one-way streets and directional restrictions. This realism is crucial for reflecting real-world road networks. When students work with directed networks, they can simulate the complexities of actual city layouts, where not all roads can be traversed in both directions. This level of detail helps them understand the practical challenges of navigation and route planning.
- Efficient Pathfinding: Directed graphs are well-known for enabling efficient algorithms for pathfinding. Algorithms such as Dijkstra's algorithm and A* search can be effectively implemented within this framework, making them essential tools for solving navigation problems. Master's students often need to find the shortest or most efficient routes between locations on a map, and directed networks streamline this process by ensuring that these algorithms account for the directionality of the roads. This efficiency in pathfinding algorithms is invaluable, both in academic assignments and in real-world applications.
- Flexibility: Directed networks offer a high degree of flexibility in representing various types of maps. Whether students are tasked with modeling a city's intricate road system or the layout of a university campus, directed networks can be adapted to suit the specific requirements of different assignment scenarios. This adaptability makes directed networks a versatile choice, allowing students to apply the same underlying principles to a wide range of map exploration challenges. They can transfer their knowledge and skills to different settings, which is beneficial for both academic learning and future professional endeavors.
Implementing Directed Networks in C
Now that we've established the advantages of using directed networks, let's discuss how to implement them in C. Here are the essential steps:
Defining Data Structures:
#include
#include
// Define the maximum number of nodes (locations) on the map
#define MAX_NODES 100
// Define the adjacency matrix
int adjacencyMatrix[MAX_NODES][MAX_NODES];
// Define an array to keep track of visited nodes during exploration
bool visited[MAX_NODES];
Initializing the Graph:
void initializeGraph(int nodes) {
for (int i = 0; i < nodes; ++i) {
visited[i] = false;
for (int j = 0; j < nodes; ++j) {
adjacencyMatrix[i][j] = 0;
}
}
}
Adding Edges:
void addEdge(int start, int end) {
// Assuming 1 represents the existence of an edge
adjacencyMatrix[start][end] = 1;
}
Depth-First Search:
void DFS(int start, int nodes) {
printf("Exploring Node %d\n", start);
visited[start] = true;
for (int i = 0; i < nodes; ++i) {
if (adjacencyMatrix[start][i] && !visited[i]) {
DFS(i, nodes);
}
}
}
Main Function:
int main() {
int nodes, edges;
printf("Enter the number of nodes and edges: ");
scanf("%d %d", &nodes, &edges);
initializeGraph(nodes);
printf("Enter the edges (start end):\n");
for (int i = 0; i < edges; ++i) {
int start, end;
scanf("%d %d", &start, &end);
addEdge(start, end);
}
int startNode;
printf("Enter the starting node for exploration: ");
scanf("%d", &startNode);
printf("Map Exploration:\n");
DFS(startNode, nodes);
return 0;
}
Depth-First Search (DFS) Algorithm
DFS is a popular algorithm for traversing graphs. It starts at the root node and explores as far as possible along each branch before backtracking. We can use DFS to find paths in our directed network.
void DFS(Graph* graph, int start, int destination) {
static int visited[MAX_NODES] = { 0 };
static int path[MAX_NODES] = { -1 };
visited[start] = 1;
Node* currentNode = graph->adjList[start];
while (currentNode != NULL) {
int neighbor = currentNode->data;
if (!visited[neighbor]) {
path[neighbor] = start;
DFS(graph, neighbor, destination);
}
currentNode = currentNode->next;
}
if (start == destination) {
printPath(path, destination);
}
}
Breadth-First Search (BFS) Algorithm
BFS is another graph traversal algorithm that explores all the vertices at the current level before moving to the next level. It can be useful for finding the shortest path between two nodes in a graph.
void BFS(Graph* graph, int start, int destination) {
int visited[MAX_NODES] = { 0 };
int path[MAX_NODES] = { -1 };
Queue* queue = createQueue(MAX_NODES);
enqueue(queue, start);
visited[start] = 1;
while (!isEmpty(queue)) {
int currentNode = dequeue(queue);
Node* neighbor = graph->adjList[currentNode];
while (neighbor != NULL) {
int nextNode = neighbor->data;
if (!visited[nextNode]) {
enqueue(queue, nextNode);
visited[nextNode] = 1;
path[nextNode] = currentNode;
}
neighbor = neighbor->next;
}
}
printPath(path, destination);
}
Testing and Debugging
Before submitting your assignment, it's essential to thoroughly test your program. Create sample maps with different configurations, and test both the DFS and BFS algorithms to ensure they work correctly. Pay special attention to edge cases and handle errors gracefully. Testing is a critical phase in software development, allowing you to catch and rectify issues before they become larger problems. By designing diverse test cases, you can validate the robustness of your code and ensure that it behaves as expected in various scenarios. Additionally, debugging plays a pivotal role in the development process, helping you identify and eliminate errors efficiently. Utilize debugging tools and techniques to trace the flow of your program, inspect variable values, and pinpoint the root causes of any anomalies. Remember that rigorous testing and effective debugging not only lead to a more polished assignment but also enhance your problem-solving skills, a valuable asset in your academic and professional journey.
Practical Applications
Simulating map exploration using a directed network has practical applications beyond university assignments. One significant avenue is the integration of classic pathfinding algorithms such as Dijkstra's Algorithm and A* Algorithm. These algorithms, explored within the simulation framework, find extensive utility in diverse fields like robotics, video games, and logistics, where determining the most efficient path between two points is critical. Moreover, the simulation's adaptability allows for seamless integration into network routing scenarios, particularly relevant in the realm of computer networks. Algorithms developed within this context can be instrumental in optimizing data packet routes, enhancing the efficiency and reliability of data transmission. Additionally, the simulation can be extended to address challenges in Geographic Information Systems (GIS), enabling the analysis and solution of real-world geographic problems. By exploring these practical applications, students not only solidify their theoretical understanding but also gain valuable insights into the versatile and impactful nature of map exploration simulations using directed networks.
GPS Navigation Systems
GPS navigation systems rely on directed networks to provide real-time directions to users. These systems have revolutionized the way people navigate the world, making travel more efficient and accessible. Understanding how these systems work can enhance your appreciation of their accuracy and efficiency. When you input a destination into your GPS device or app, it's conducting complex calculations on a directed graph of roadways, considering one-way streets, traffic conditions, and real-time updates. The knowledge gained from working with directed networks in assignments can directly translate to a deeper understanding of GPS technology. This understanding is not only beneficial for everyday navigation but can also lead to innovations in GPS systems and related technologies.
Social Network Analysis
Another practical application of directed networks lies in the realm of social network analysis. Social networks, both online and offline, can be effectively represented as directed graphs. Understanding how information flows within these networks, who the influencers are, and how individuals and groups are connected can be crucial in various fields. Whether it's in marketing, sociology, or even national security, the ability to analyze and interpret social network data is invaluable. By working with directed networks in academic assignments, you're building the skills necessary to conduct advanced social network analyses. This knowledge can lead to insights that have far-reaching implications in the digital age. Whether you're studying the spread of information on a social media platform or the interactions within an organization, directed network skills are highly transferable and beneficial.
Conclusion
The simulation of map exploration using directed networks not only aids master's students in their academic assignments but also opens doors to a plethora of real-world applications. From enhancing GPS navigation systems to empowering social network analysis, the skills honed in this pursuit are versatile and in-demand. As students delve into the intricacies of directed networks, they are not merely mastering algorithms; they are equipping themselves to tackle the complexities of modern technology and society. This knowledge is not confined to the classroom but extends into industries and domains where directed networks play a pivotal role. By recognizing the broader practical applications of this approach, students can embrace the challenges of their assignments with a profound sense of purpose, knowing that their acquired skills hold the potential to shape and improve our rapidly evolving world.